# Standards chompkamekbloopers
# Naming conventions
# General Files and Folders
Kebab case. Kebab case combines words by replacing each space with a dash (-).
.
└─ folder-name
└─ file-name.extension
# Vue Components Files and Folders
Pascal case. Pascal case combines words by capitalizing all words (even the first word) and removing the space.
Don't save characteres, make it descriptive and intuitive!
.
├─ _components
│ ├─ PurchasesForm
│ │ ├─ PurchasesForm.vue
│ │ ├─ PurchasesForm.stories.js
│ │ └─ PurchasesForm.spec.js
│ └─ RefundsForm
│ ├─ RefundsForm.vue
│ ├─ RefundsForm.stories.js
│ └─ RefundsForm.spec.js
├─ ViewPurchasesList.vue # list of all purchases
├─ ViewPurchasesDetails.vue # details of a purchase
├─ ViewPurchasesEdit.vue # edit a purchase
├─ ViewPurchasesRefundsList.vue # list of refunds to a specific purchase
├─ ViewPurchasesRefundsDetails.vue # details of a refund
├─ ViewPurchasesRefundsCreate.vue # create a refund to a specific purchase
└─ ViewRefunds.vue # list of all refunds
# Constants
Snake case. Snake case combines words by replacing each space with an underscore (_) and, in the all caps version, all letters are capitalized.
export const CREATE = 'create'
export const EDIT = 'edit'
export const DOWNLOAD = 'download'
export const CANCEL = 'cancel'
export const SUBMIT = 'submit'
export const DELETE = 'delete'
# Vuex Mutations
function <ACTION>_<MODULE>_<STATUS>() {}
<ACTION>
could be GET, CREATE, EDIT, DELETE, ...<MODULE>
could be USERS, ROLES, PAYMENT_METHODS, ...<STATUS>
could be:- PENDING - when something is being processed. Usually used to set loadings and clear errors;
- SUCCESS - when something was successfully processed. Usually used to stop loadings and set informating in the store;
- ERROR - when something went wrong. Usually used to stop loadings and set API errors in the store.
function GET_USERS_PENDING() {}
function GET_USERS_SUCCESS() {}
function GET_USERS_ERROR() {}
function CREATE_USERS_PENDING() {}
function CREATE_USERS_SUCCESS() {}
function CREATE_USERS_ERROR() {}
# Linting and Prettier
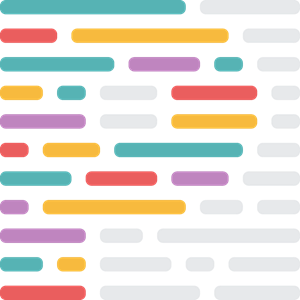
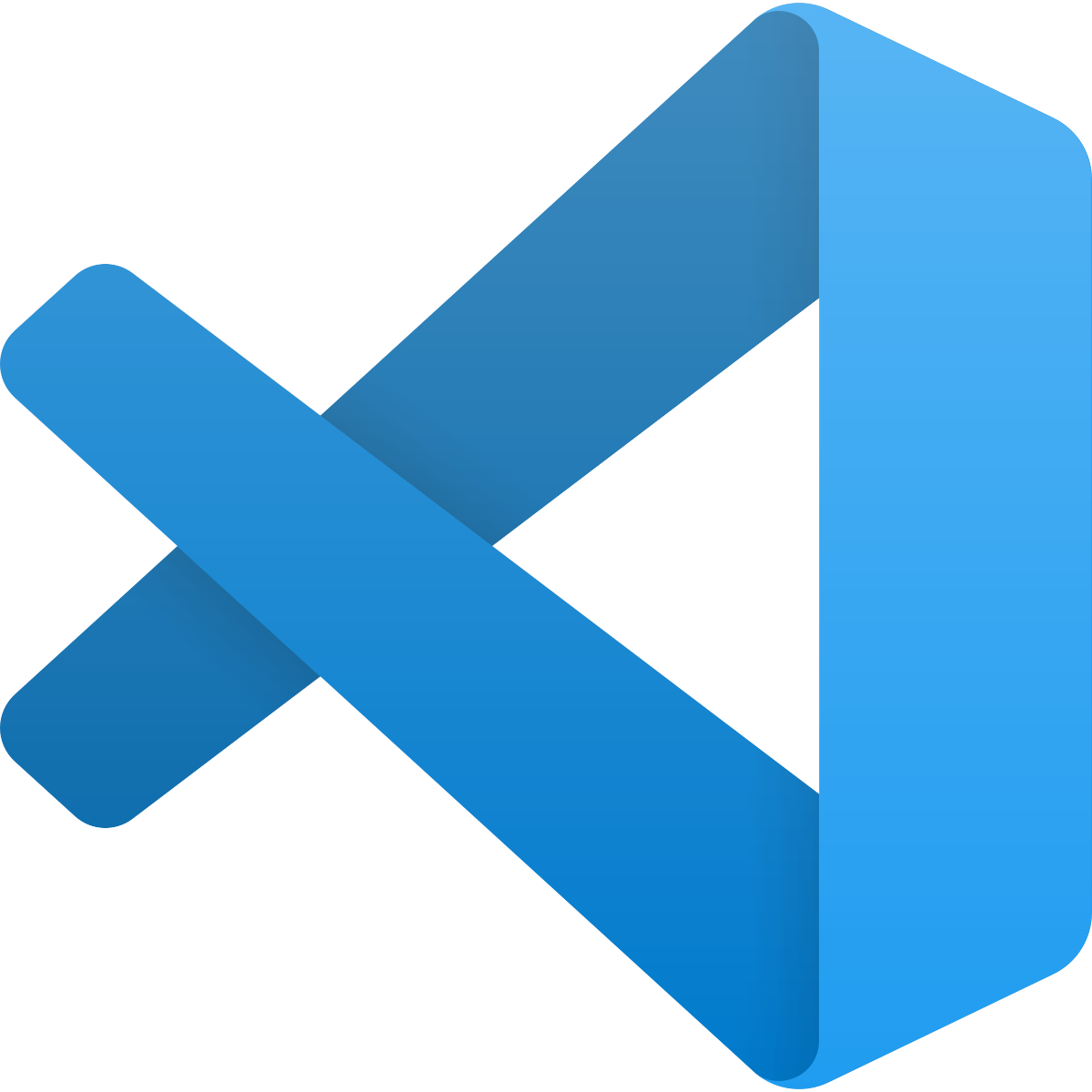
Standards are important to allow everyone to know what should be expected in the projects. To enforce the standards we use ESLint (opens new window) and Prettier (opens new window). Visual Studio Code (opens new window) has plugins that give us information about lint errors and fix them, when possible, on save. Linting also runs when we do git commit
allowing only to send code following our standards.
Vue.js provides a eslint plugin (opens new window) that help us with it specific features. We follow the Priority C: Recommended.