# Integration Tests chompkamek
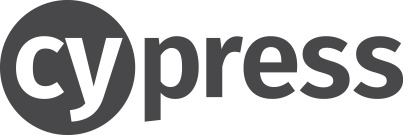
To do integration tests it is used Cypress (opens new window).
These tests are placed inside the folder tests/e2e/specs
in the root of the project. Here we create a folder for the module to test and inside the folder the tests itself.
The naming of the files can follow two approaches:
<module>_<page>.test.js
<module>_<action>.test.js
Each page of the application must have a file
- purchases-list.test.js
- purchases-details.test.js
- purchases-refunds-list.test.js
- purchases-refunds-details.test.js
Each action that can be made in the module must have its own test file:
- purchases-refunds-create.test.js
- purchases-refunds-approve.test.js
Following this approach, the files won't become too big and it will be easier to find what we are searching for.
# Forms
Each module that as a form should have it's own test file
- purchases-refunds-form.test.js
# Validations
The tests should cover every validation done on the frontend.
Best Practices
- The mandatory fields should all be tested at once
describe('Mandatory fields', () => {
it('"name", "description" and "file" are mandatory', () => {
cy.visit(visitUrl)
cy.submitForm()
cy.testFieldError({
selector: topUpsSelectors.nameError,
value: 'Name is mandatory'
})
cy.testFieldError({
selector: topUpsSelectors.descriptionError,
value: 'Description is mandatory'
})
cy.testFieldError({
selector: topUpsSelectors.fileError,
value: 'File is mandatory'
})
})
})
- The other validations of each field should be grouped
describe('Amount rules', () => {
it('"amount" must be equal or lower than the available balance', () => {
cy.visit(visitUrl).wait('@getWalletAccounts')
cy.typeInTextInput({
selector: b2cCreditsSelectors.amountInput,
value: '150'
})
cy.submitForm()
cy.testFieldError({
selector: b2cCreditsSelectors.amountError,
value: 'Amount must be equal or lower than 123€'
})
})
it('"amount" must be higher than 0', () => {
cy.visit(visitUrl).wait('@getWalletAccounts')
cy.typeInTextInput({
selector: b2cCreditsSelectors.amountInput,
value: '0'
})
cy.submitForm()
cy.testFieldError({
selector: b2cCreditsSelectors.amountError,
value: 'Amount must be higher than 0'
})
})
})
# Utility Commands
Types a number or a string on text input's
cy.typeInTextInput({selector, value})
Select the "position" item on dropdown
cy.selectItemOnDropdown({ inputSelector, optionSelector, position = 0 })
Check if the input is displaying the correct error
cy.testFieldError({ selector, value })
Test that the input length don't exceed the max length
cy.testFieldMaxLength({ selector, maxLength, isNumber = false })
Submit the form
cy.submitForm()
WARNING
- Tests are comparing the english error messages!
- Tests for the form validations were not done with unit tests due to js dom limitations.